Searching: Understanding Linear and Sequential Search in ArrayLists
Introduction to Searching
Searching is a fundamental operation in programming that allows us to determine whether a specific element exists in a data structure and, if it does, identify its position. This operation is vital for numerous applications, ranging from simple data retrieval to complex algorithmic processes.
One of the simplest and most commonly used searching techniques is linear search, also known as sequential search. This algorithm iterates through each element in the collection one by one until the target element is found or the end of the collection is reached. While it may not be the most efficient searching method, linear search is highly versatile and straightforward, making it an excellent starting point for learning about searching.
What is Linear/Sequential Search?
Linear or sequential search is an algorithm that:
Iterates through each element in the data structure sequentially.
Compares each element to the target value.
Returns the index of the element if a match is found.
Returns
-1
if the element is not found in the collection.
This algorithm works for both ArrayLists and regular arrays, making it a versatile tool for developers.
Linear Search in ArrayLists
ArrayLists are dynamic data structures in Java that allow us to store and manipulate a collection of objects. Searching within an ArrayList is a common task, and linear search is the simplest method for accomplishing this.
Implementation for ArrayLists
Below is the Java implementation of linear search for an ArrayList:
/**
* Performs a linear search on an ArrayList to find the target element.
* @param array The ArrayList to search through.
* @param n The target element to find.
* @return The index of the target element, or -1 if not found.
*/
public static int linearSearch(ArrayList<Integer> array, int n) {
for (int i = 0; i < array.size(); i++) {
if (array.get(i) == n) {
return i;
}
}
return -1; // Element not found
}
How It Works
A
for
loop iterates through the ArrayList from index0
toarray.size() - 1
.The
get()
method retrieves each element for comparison.If a match is found, the method returns the index of the element.
If no match is found by the end of the loop, the method returns
-1
.
Linear Search in Integer Arrays
Linear search can also be applied to standard integer arrays. While the underlying principle remains the same, the implementation differs slightly due to the differences in accessing elements between arrays and ArrayLists.
Implementation for Integer Arrays
/**
* Performs a linear search on an integer array to find the target element.
* @param array The array to search through.
* @param n The target element to find.
* @return The index of the target element, or -1 if not found.
*/
public static int linearSearch(int[] array, int n) {
for (int i = 0; i < array.length; i++) {
if (array[i] == n) {
return i;
}
}
return -1; // Element not found
}
Key Differences Between ArrayLists and Arrays
Accessing Elements:
In ArrayLists, elements are accessed using the
get()
method.In arrays, elements are accessed directly using square brackets (
[]
).
Size Property:
For ArrayLists, use the
size()
method.For arrays, use the
length
property.
Advantages of Linear Search
Simplicity:
Easy to understand and implement.
No additional setup or data structure modifications are needed.
Versatility:
Works on both sorted and unsorted collections.
Applicable to various data structures, including arrays, ArrayLists, and linked lists.
Dynamic Collection Compatibility:
Ideal for dynamic collections like ArrayLists where the size is not fixed.
Disadvantages of Linear Search
Inefficiency for Large Collections:
The time complexity is O(n), where
n
is the number of elements. This makes linear search inefficient for large datasets.
No Early Exit for Sorted Data:
Even if the data is sorted, linear search does not take advantage of this property.
Not Optimal for Frequent Searches:
If searching is a frequent operation, more advanced algorithms like binary search (covered in Unit 10) may be more suitable.
Practical Applications of Linear Search
Data Retrieval:
Searching for specific items in shopping carts, user profiles, or dynamic lists.
Validation Checks:
Ensuring a user input exists within a predefined set of acceptable values.
Error Detection:
Locating erroneous or outlier data in a collection.
Best Practices for Linear Search
Optimize for Small Collections:
Use linear search only for smaller datasets where the inefficiency is negligible.
Use Early Exits:
Return the result as soon as the element is found to avoid unnecessary iterations.
Enhance Readability:
Comment your code to make it clear that linear search is being used and why it was chosen.
Conclusion
Searching is a crucial operation in any programming task, and linear search provides a straightforward introduction to this concept. While it may not be the most efficient algorithm for large datasets, its simplicity and versatility make it an essential tool for small to medium-sized collections.
Understanding how to implement and optimize linear search for both ArrayLists and arrays lays the groundwork for exploring more advanced searching techniques, such as binary search. By mastering these fundamentals, you can ensure your programs are robust and capable of handling various data retrieval scenarios.
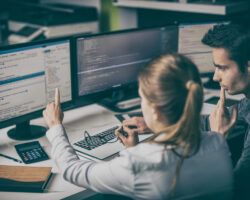
50 Highly Trending FAQs About Searching with Detailed Answers
1. What is Searching in Computer Science?
Searching is the process of finding a specific element or set of elements in a data structure, such as arrays, lists, or databases, based on a given key or value.
2. What Are the Types of Searching Algorithms?
Linear Search
Binary Search
Hash-Based Search
Depth-First Search (DFS)
Breadth-First Search (BFS)
3. What is Linear Search?
Linear Search sequentially checks each element in a list until the target element is found or the list ends. It works well for small, unsorted datasets.
4. What is Binary Search?
Binary Search divides a sorted dataset into halves, repeatedly eliminating one half based on comparisons until the target is found. It has a time complexity of O(log n).
5. When to Use Linear Search?
Linear Search is used when:
The dataset is small.
The dataset is unsorted.
6. When to Use Binary Search?
Binary Search is effective for:
Large datasets.
Sorted data structures.
7. How to Implement Linear Search in Java?
int linearSearch(int[] arr, int target) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target) return i;
}
return -1;
}
8. How to Implement Binary Search in Python?
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
9. What is the Time Complexity of Linear Search?
The time complexity of Linear Search is O(n), where n is the number of elements in the dataset.
10. What is the Time Complexity of Binary Search?
The time complexity of Binary Search is O(log n), where n is the number of elements in the dataset.
11. What is Depth-First Search (DFS)?
DFS is a graph traversal algorithm that explores as far down a branch as possible before backtracking.
12. What is Breadth-First Search (BFS)?
BFS is a graph traversal algorithm that explores all neighbors at the current depth before moving to the next depth.
13. How is Searching Used in Databases?
Searching in databases often involves:
Querying tables using SQL.
Indexing for faster lookups.
14. What is Interpolation Search?
Interpolation Search improves on Binary Search by estimating the position of the target based on the dataset’s distribution. It works best with uniformly distributed data.
15. What is Exponential Search?
Exponential Search finds a range where the target might exist and then performs Binary Search within that range. It is useful for unbounded or infinite datasets.
16. What is a Hash-Based Search?
Hash-Based Search uses a hash function to map keys to specific indices, allowing O(1) average-time complexity for lookups.
17. What is a Sentinel in Linear Search?
A sentinel is a special value added to the end of a dataset to simplify and speed up the Linear Search by eliminating the need for boundary checks.
18. What is Ternary Search?
Ternary Search splits the dataset into three parts and eliminates two-thirds of the data at each step. It is similar to Binary Search but less common.
19. How is Searching Implemented in Hash Tables?
Hash tables use a hash function to compute the index for storing and retrieving elements, offering O(1) average lookup time.
20. What is Jump Search?
Jump Search skips a fixed number of elements (jump size) and then performs Linear Search in the identified block. It works well for sorted datasets.
21. What is the Role of Indexing in Searching?
Indexing speeds up searching by creating a separate data structure (index) that maps keys to their locations in the main dataset.
22. How to Perform Searching in Strings?
Use methods like contains()
in Java:
String str = "hello";
boolean found = str.contains("he");
23. What is Pattern Matching in Searching?
Pattern matching involves searching for substrings within strings using algorithms like KMP or Boyer-Moore.
24. What is Binary Search Tree (BST) Searching?
In a BST, elements are searched by recursively comparing the target with the node values, starting from the root.
25. What is Trie Searching?
Trie is a tree-like data structure for searching strings or prefixes efficiently, often used in autocomplete systems.
26. What is the Best Search Algorithm for Large Datasets?
Binary Search or Hash-Based Search, depending on whether the dataset is sorted or hashable.
27. What is the Difference Between Sequential and Parallel Searching?
Sequential Searching: Processes elements one at a time.
Parallel Searching: Divides the dataset into parts and searches them concurrently.
28. What is Fuzzy Searching?
Fuzzy Searching finds approximate matches, often using algorithms like Levenshtein Distance or edit distance calculations.
29. What is Binary Indexed Tree (BIT) Searching?
BIT is a data structure for efficient searching and manipulation of prefix sums.
30. How to Implement Searching in Graphs?
Use DFS or BFS for graph-based searching.
31. What is Space Complexity of Searching Algorithms?
Linear Search: O(1)
Binary Search: O(1)
DFS/BFS: O(V + E), where V is vertices and E is edges.
32. What is Online Searching?
Online Searching processes data as it arrives, without requiring the entire dataset beforehand.
33. What is Offline Searching?
Offline Searching requires the complete dataset to be available before starting the search.
34. What is A* Search Algorithm?**
A* is a heuristic-based algorithm used in pathfinding and graph traversal, balancing cost and estimated distance to the target.
35. What is Uniform Cost Search?
Uniform Cost Search expands the least-cost node first and is used in weighted graphs.
36. What is Searching in Artificial Intelligence?
AI searching involves algorithms like A*, Greedy Search, and Minimax to explore possibilities and make decisions.
37. What is Multidimensional Searching?
Searching across multiple dimensions (e.g., 2D or 3D arrays) using algorithms like Range Trees or KD Trees.
38. How to Search in Circular Arrays?
Use modular arithmetic to wrap around indices:
index = (currentIndex + step) % len(array)
39. What is Distributed Searching?
Distributed Searching divides the dataset across multiple machines and processes them in parallel.
40. What is the Role of Hash Functions in Searching?
Hash functions map keys to indices, enabling O(1) average-time complexity for lookups in hash tables.
41. What is Nearest Neighbor Search?
Nearest Neighbor Search finds the closest point(s) to a given target in a multidimensional space.
42. What is Exact Searching?
Exact Searching looks for elements that match the query exactly.
43. What is Approximate Searching?
Approximate Searching identifies results that are similar but not identical to the query.
44. What is Keyword Searching?
Keyword Searching finds text or data matching specific keywords, commonly used in search engines.
45. What is Weighted Searching?
Weighted Searching assigns weights to elements or paths, optimizing for the highest or lowest total weight.
46. How to Search in Rotated Sorted Arrays?
Use a modified Binary Search to account for rotation.
47. What is HashMap Searching?
HashMap Searching retrieves values in O(1) average time by key lookups.
48. What is Full-Text Searching?
Full-Text Searching indexes entire documents to quickly find matches for search queries.
49. What is Range Searching?
Range Searching identifies all elements within a specific range in a dataset.
50. What is Adaptive Searching?
Adaptive Searching adjusts its strategy based on the dataset’s characteristics, like size or distribution.